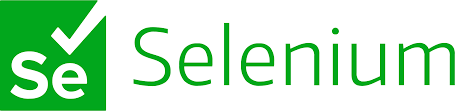
Here are the top 50 interview questions and answers for Selenium 4, covering a range of topics including new features, basic concepts, and advanced usage. These questions are designed to help you prepare effectively for a Selenium interview.
Basic Selenium Questions
- What is Selenium?
- Answer: Selenium is an open-source tool used for automating web browsers. It provides a suite of tools for different browser automation tasks.
- What are the components of Selenium?
- Answer: Selenium IDE, Selenium WebDriver, Selenium Grid, and Selenium Standalone Server.
- What is Selenium WebDriver?
- Answer: WebDriver is a web automation framework that allows you to execute your tests against different browsers.
- What is the difference between Selenium 3 and Selenium 4?
- Answer: Selenium 4 introduces W3C standardization, enhanced Selenium Grid, better IDE, new Window and Tab management, and relative locators.
- How do you install Selenium WebDriver?
- Answer: By including the Selenium library in your project dependencies, e.g., using Maven for Java.
Selenium 4 Specific Questions
- What are the new features in Selenium 4?
- Answer: Native Chrome DevTools Protocol support, new relative locators, improved Selenium Grid, better documentation and support for modern web testing needs.
- Explain the use of relative locators in Selenium 4.
- Answer: Relative locators allow you to locate elements in relation to other elements (e.g., above, below, toLeftOf, toRightOf).
- What is the W3C WebDriver standard in Selenium 4?
- Answer: It standardizes the WebDriver API to enhance compatibility across different browsers.
- How do you perform browser tab management in Selenium 4?
- Answer: Using
newWindow(WindowType.TAB)
andnewWindow(WindowType.WINDOW)
methods.
- How has Selenium Grid improved in Selenium 4?
- Answer: The new Selenium Grid comes with Docker support, an improved UI, and better cloud integration.
WebDriver Questions
- What are WebDriver wait commands?
- Answer: WebDriver wait commands include implicit wait, explicit wait, and fluent wait to handle synchronization issues.
- How do you handle alerts in Selenium?
- Answer: Using the
Alert
interface with methods likeaccept()
,dismiss()
,getText()
, andsendKeys()
.
- Answer: Using the
- Explain the difference between
findElement()
andfindElements()
.- Answer:
findElement()
returns a single WebElement, whereasfindElements()
returns a list of WebElements.
- Answer:
- How do you switch between frames in Selenium?
- Answer: Using
driver.switchTo().frame()
with index, name, or WebElement.
- Answer: Using
- How do you handle multiple windows in Selenium?
- Answer: Using
driver.switchTo().window(windowHandle)
to switch control between windows.
- Answer: Using
Advanced Questions
- How can you handle dynamic web elements in Selenium?
- Answer: By using dynamic locators, explicit waits, and JavaScriptExecutor for direct interactions.
- What is the use of JavaScriptExecutor in Selenium?
- Answer: JavaScriptExecutor allows executing JavaScript code within the context of the browser.
- How do you upload a file using Selenium WebDriver?
- Answer: Using the
sendKeys()
method on a file input element to upload files.
- Answer: Using the
- Explain how to use Selenium Grid.
- Answer: Selenium Grid allows you to run tests on different machines against different browsers in parallel.
- What are the benefits of using Page Object Model (POM)?
- Answer: POM promotes code reusability, maintainability, and separation of test logic from the UI elements.
Coding and Implementation Questions
- Write a code to launch Chrome browser using WebDriver.
WebDriver driver = new ChromeDriver(); driver.get("https://www.example.com");
- How do you maximize the browser window in Selenium?
- Answer: Using
driver.manage().window().maximize();
.
- Answer: Using
- How can you take a screenshot in Selenium?
- Answer: Using
TakesScreenshot
interface andgetScreenshotAs
method.
- Answer: Using
- Explain the use of
Actions
class in Selenium.- Answer:
Actions
class is used for handling complex user interactions like drag-and-drop, hover, etc.
- Answer:
- How do you implement an explicit wait?
java WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Miscellaneous Questions
- What is the difference between assert and verify commands in Selenium?
- Answer:
assert
stops test execution on failure, whileverify
continues execution even if the check fails.
- Answer:
- How do you handle pop-ups in Selenium?
- Answer: By using alert handling methods or switching to the pop-up window if it’s a new browser window.
- What are some common exceptions in Selenium WebDriver?
- Answer: NoSuchElementException, TimeoutException, StaleElementReferenceException, WebDriverException.
- How do you handle dropdowns in Selenium?
- Answer: Using the
Select
class to interact with dropdown elements.
- Answer: Using the
- Explain the use of DesiredCapabilities in Selenium.
- Answer: DesiredCapabilities are used to set the properties of the WebDriver during the test execution.
Advanced Scripting Questions
- How can you perform a drag and drop operation in Selenium?
Actions actions = new Actions(driver); WebElement source = driver.findElement(By.id("source")); WebElement target = driver.findElement(By.id("target")); actions.dragAndDrop(source, target).perform();
- How do you work with cookies in Selenium?
- Answer: Using
driver.manage().getCookies()
,driver.manage().addCookie()
, anddriver.manage().deleteCookie()
methods.
- Answer: Using
- What is the FluentWait class in Selenium?
- Answer: FluentWait is used for handling dynamic waits with specific polling time and ignoring specific exceptions.
- How do you handle SSL certificates in Selenium?
- Answer: By using DesiredCapabilities to accept insecure SSL certificates.
- Explain the use of WebDriver Event Listener.
- Answer: WebDriver Event Listeners are used to track events triggered by WebDriver during test execution for logging or other purposes.
Practical Implementation Questions
- How do you automate a login page in Selenium?
driver.findElement(By.id("username")).sendKeys("user"); driver.findElement(By.id("password")).sendKeys("password"); driver.findElement(By.id("loginButton")).click();
- How can you validate broken links in a webpage using Selenium?
- Answer: By extracting all links and verifying the HTTP response codes using an HTTP client library.
- How do you run Selenium tests in headless mode?
- Answer: By configuring the WebDriver to run in headless mode, e.g., using
ChromeOptions
orFirefoxOptions
.
- Answer: By configuring the WebDriver to run in headless mode, e.g., using
- What is the use of the WebDriverWait class?
- Answer: WebDriverWait is used for applying explicit waits to synchronize the execution flow.
- How do you perform database testing using Selenium?
- Answer: By using JDBC to connect to the database and execute SQL queries to validate data.
Selenium Integration Questions
- How do you integrate Selenium with CI/CD tools like Jenkins?
- Answer: By creating Jenkins jobs to run Selenium tests as part of the build process and generating test reports.
- Explain the use of TestNG with Selenium.
- Answer: TestNG provides advanced features like annotations, grouping, parallel execution, and detailed reporting for Selenium tests.
- How do you handle JavaScript alerts in Selenium?
- Answer: Using the
Alert
interface with methods such asaccept()
,dismiss()
,getText()
, andsendKeys()
.
- Answer: Using the
- How can you achieve cross-browser testing using Selenium?
- Answer: By configuring WebDriver to run tests on different browsers like Chrome, Firefox, Safari, and Edge.
- What are some best practices for writing Selenium tests?
- Answer: Using POM, keeping locators in separate files, writing reusable methods, using waits appropriately, and maintaining readability.
Framework and Design Questions
- What is the Page Factory in Selenium?
- Answer: Page Factory is a way to implement the Page Object Model with support for lazy initialization and other features.
- How do you handle AJAX elements in Selenium?
- Answer: By using explicit waits to wait for the AJAX elements to be loaded and interactable.
- Explain the Singleton design pattern in Selenium framework.
- Answer: Singleton pattern ensures only one instance of the WebDriver is created and reused across the test suite.
- How do you manage test data in Selenium tests?
- Answer: By using external files (CSV, Excel, JSON) or databases to manage and provide test data.
- **What is the role of an
Here are the remaining questions and answers for the top 50 interview questions on Selenium 4, continuing from where we left off:
Framework and Design Questions (Continued)
- What is the role of an automation framework in Selenium testing?
- Answer: An automation framework provides a structured environment for writing and managing test cases efficiently. It includes guidelines for coding standards, handling test data, managing test execution, generating reports, and integrating with other tools and technologies.
Additional Practical and Advanced Questions
- How do you handle Captcha in Selenium?
- Answer: Captchas are designed to be difficult for bots. Typically, you might use third-party services or manual intervention to handle Captchas in test scenarios.
- What are the different types of waits available in Selenium WebDriver?
- Answer: Implicit Wait, Explicit Wait, and Fluent Wait.
- Explain the use of
WebDriverWait
in Selenium.- Answer:
WebDriverWait
is used for applying explicit waits. It waits until a specified condition is met before proceeding with the test execution.
- Answer:
- How do you handle file downloads in Selenium?
- Answer: By setting browser preferences and handling the download location through WebDriver options or using a third-party library.
- What are some challenges faced in Selenium automation?
- Answer: Handling dynamic elements, managing synchronization issues, dealing with browser compatibility, and handling CAPTCHA or other forms of security.
- How do you verify the tooltip text in Selenium?
- Answer: By using the
getAttribute("title")
method to retrieve the tooltip text.
- Answer: By using the
- How can you achieve data-driven testing in Selenium?
- Answer: Using frameworks like TestNG or JUnit along with external data sources such as Excel, CSV, or databases to drive test cases with different data sets.
- What is the difference between
getWindowHandles()
andgetWindowHandle()
?- Answer:
getWindowHandles()
returns a set of all window handles, whereasgetWindowHandle()
returns the current window handle.
- Answer:
- How do you handle web tables in Selenium?
- Answer: By locating the table element and iterating through its rows and columns to extract data or perform actions.
- How do you perform mouse hover actions in Selenium?
- Answer: Using the
Actions
class to move to the desired element and perform a hover action.
- Answer: Using the
Continuous Integration and Reporting Questions
- How do you generate test reports in Selenium?
- Answer: Using TestNG or JUnit built-in reporting, or integrating with reporting tools like Allure, ExtentReports, or custom HTML reports.
- Explain how you can integrate Selenium with Jenkins for continuous integration.
- Answer: By configuring Jenkins to pull code from the version control system, executing Selenium tests, and generating test reports.
- What are some common tools used for reporting in Selenium?
- Answer: Allure, ExtentReports, TestNG, JUnit, and custom report generators.
- How do you manage browser compatibility issues in Selenium?
- Answer: By running tests on different browsers and using WebDriver capabilities to handle browser-specific issues.
- How can you execute Selenium tests in parallel?
- Answer: Using TestNG parallel execution, Selenium Grid, or Docker containers.
Advanced and Scenario-Based Questions
- How do you handle AJAX calls in Selenium?
- Answer: By using explicit waits to wait for elements to be available or for conditions to be met after an AJAX call.
- What is the use of the
Actions
class in Selenium?- Answer: The
Actions
class is used to handle advanced user interactions like drag and drop, hover, click and hold, etc.
- Answer: The
- Explain the
Select
class in Selenium and its usage.- Answer: The
Select
class is used for interacting with dropdown elements in a web page, providing methods to select options by index, value, or visible text.
- Answer: The
- How do you capture network traffic in Selenium?
- Answer: Using tools like BrowserMob Proxy or Selenium 4’s Chrome DevTools Protocol integration to intercept and inspect network traffic.
- What is the importance of maintaining test scripts in Selenium?
- Answer: Maintaining test scripts ensures that tests are up-to-date, reliable, and effective in identifying issues, leading to better software quality and easier maintenance.
Debugging and Optimization Questions
- How do you debug Selenium test scripts?
- Answer: By using breakpoints, step execution, and debugging tools provided by the IDE (e.g., Eclipse, IntelliJ IDEA).
- What are some common issues in Selenium tests and how do you resolve them?
- Answer: Common issues include element not found exceptions, synchronization issues, and browser compatibility problems. These can be resolved using waits, updating locators, and cross-browser testing strategies.
- How do you optimize Selenium test execution?
- Answer: By using parallel execution, headless browsers, efficient locators, and minimizing unnecessary waits or operations.
- Explain the concept of headless browsers and their use in Selenium.
- Answer: Headless browsers allow running tests without a GUI, which is faster and useful for CI environments. Examples include Headless Chrome and Headless Firefox.
- How do you handle stale element exceptions in Selenium?
- Answer: By re-locating the element or using explicit waits to ensure the element is present in the DOM.
Scenario-Based Questions
- How would you automate a scenario where you need to perform a login, navigate to a specific section, and verify a specific element?
- Answer: Write a script to locate and interact with the login elements, perform the login, navigate to the section using navigation elements, and use assertions to verify the element.
- Describe a situation where you need to handle multiple iframes within a page.
- Answer: Switch to each iframe using
driver.switchTo().frame()
and perform the required actions or validations within each iframe context.
- Answer: Switch to each iframe using
- How do you validate the sorting functionality of a table column in Selenium?
- Answer: Extract the column data, sort it using the desired sorting algorithm, and compare it with the data in the web table to verify correctness.
- Explain how to handle browser notifications in Selenium.
- Answer: Using browser-specific options like ChromeOptions or FirefoxOptions to disable or handle notifications.
- How would you automate testing for responsive web design in Selenium?
- Answer: By resizing the browser window to different screen resolutions and verifying the layout and functionality for each resolution.
Miscellaneous Questions
- How do you manage test dependencies in Selenium?
- Answer: Using TestNG or JUnit annotations to define dependencies between test methods or using dependency injection frameworks.
- What is the role of Maven or Gradle in Selenium projects?
- Answer: Maven and Gradle manage project dependencies, build lifecycle, and provide a standardized project structure.
- How do you handle date pickers in Selenium?
- Answer: By interacting with the date picker elements directly or using JavaScript to set date values.
- Explain the use of the
WebDriverWait
class in Selenium.- Answer:
WebDriverWait
applies explicit waits, allowing you to wait for specific conditions to be met before proceeding with the test execution.
- Answer:
- How do you handle browser zoom level issues in Selenium?
- Answer: By setting the browser zoom level to 100% using browser-specific settings or WebDriver options.
Framework and Design Questions (Continued)
- What is the difference between Page Object Model (POM) and Page Factory?
- Answer: POM is a design pattern that creates object repositories for web elements, while Page Factory is an implementation of POM with lazy initialization and other enhancements.
- Explain how you handle data-driven testing in Selenium.
- Answer: Using frameworks like TestNG or JUnit with external data sources to drive test cases with different data sets.
- How do you manage environment-specific configurations in Selenium?
- Answer: By using configuration files or environment variables to store environment-specific settings and accessing them in test scripts.
- Describe the role of annotations in TestNG for Selenium testing.
- Answer: Annotations in TestNG, like
@BeforeMethod
,@AfterMethod
,@Test
, etc., define test setup, teardown, and execution flow.
- Answer: Annotations in TestNG, like
- How do you handle dynamic web elements in Selenium?
- Answer: By using dynamic locators, explicit waits, and JavaScriptExecutor to handle elements that change frequently.
Best Practices and Optimization Questions
- What are some best practices for writing maintainable Selenium tests?
- Answer: Use Page Object Model, maintain a clear structure, use meaningful names for locators and methods, implement proper error handling, and regularly review and refactor test scripts.
- How do you ensure the reliability of Selenium tests?
- Answer: By using robust locators, applying appropriate waits, handling exceptions gracefully, and running tests regularly to identify and fix flakiness.
- Explain the use of browser profiles in Selenium.
- Answer: Browser profiles allow configuring browser settings, extensions, and preferences, which can be reused during test execution.
- How do you handle complex user interactions in Selenium?
- Answer: Using the
Actions
class to simulate
- Answer: Using the
Continuing with the advanced Selenium 4 interview questions and answers:
Advanced and Scenario-Based Questions (Continued)
- Explain how you handle complex user interactions in Selenium.
- Answer: Using the
Actions
class to simulate complex user interactions like drag and drop, double click, right click, and mouse hover. For example:
Actions actions = new Actions(driver); WebElement element = driver.findElement(By.id("elementId")); actions.moveToElement(element).click().build().perform();
- Answer: Using the
- How do you handle file downloads in headless mode?
- Answer: Configure the browser’s download preferences programmatically to specify the download location and automatically handle download prompts.
- How do you manage dependencies between test methods in Selenium?
- Answer: Using TestNG annotations like
@DependsOnMethods
to specify dependencies between test methods, ensuring that certain tests run only if others have passed.
- Answer: Using TestNG annotations like
- How do you execute JavaScript commands in Selenium?
- Answer: Using the
JavascriptExecutor
interface to run JavaScript commands within the browser context:
JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("return document.title;");
- Answer: Using the
- How do you validate text present on a web page in Selenium?
- Answer: Use the
getText()
method to retrieve the text from a web element and validate it using assertions:
String actualText = driver.findElement(By.id("elementId")).getText(); Assert.assertEquals(actualText, "Expected Text");
- Answer: Use the
- Describe a scenario where you need to handle a web element that is not immediately visible.
- Answer: Use explicit waits to wait for the element to become visible before interacting with it:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId"))); element.click();
- How do you perform mobile testing with Selenium?
- Answer: By integrating Selenium with Appium, which allows you to automate mobile applications on Android and iOS.
- How do you manage test execution order in Selenium?
- Answer: By using TestNG annotations and XML configuration files to control the order of test execution.
- Explain the difference between
driver.quit()
anddriver.close()
.- Answer:
driver.close()
closes the current browser window, whiledriver.quit()
closes all browser windows and ends the WebDriver session.
- Answer:
- How do you handle SSL certificate errors in Selenium?
- Answer: Configure the WebDriver to accept untrusted certificates using browser-specific options like:
ChromeOptions options = new ChromeOptions(); options.setAcceptInsecureCerts(true); WebDriver driver = new ChromeDriver(options);
- What are some ways to improve the performance of Selenium tests?
- Answer: Use headless browsers, parallel execution, optimized locators, minimize the use of waits, and keep the test scripts clean and modular.
Miscellaneous Questions (Continued)
- How do you integrate Selenium with other testing frameworks like Cucumber?
- Answer: By writing test steps in Gherkin syntax and implementing step definitions using Selenium WebDriver within a Cucumber framework.
- How do you perform browser compatibility testing using Selenium?
- Answer: By running the same set of tests across different browsers and browser versions using Selenium Grid or cross-browser testing tools like BrowserStack or Sauce Labs.
- Explain how to use Selenium with BDD (Behavior Driven Development).
- Answer: Using tools like Cucumber to write test cases in a natural language format and Selenium WebDriver to implement the steps, promoting collaboration between technical and non-technical team members.
- How do you handle AJAX calls using Selenium WebDriver?
- Answer: Use explicit waits to wait for AJAX elements to load or for specific conditions to be met:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); wait.until(ExpectedConditions.elementToBeClickable(By.id("ajaxElementId")));
- How do you ensure your Selenium tests are maintainable?
- Answer: Use design patterns like Page Object Model, keep your test code clean and modular, regularly refactor tests, and document your test cases.
By preparing for these questions and understanding the answers, you’ll be well-equipped to handle a variety of topics in Selenium 4 interviews.